Making Phunky
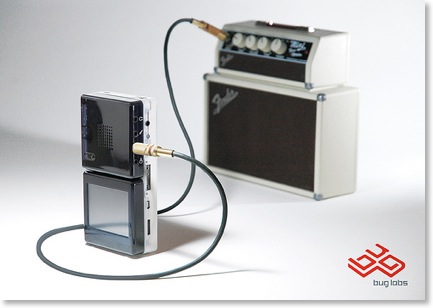
Bug Labs recently came out with their BUGsound module. I made a fun little application for it using the BUGmotion’s accelerometer stream to pick from a set of samples. The accelerometer is a 3-axis, so I assigned each axis 3 samples to choose from; the application then chooses one of those 3 samples based on that axis’ current reading and mixes the chosen samples for all the axes together. I picked the sample changeover point for each axis based on experimentation by moving the BUG around while printing out the accelerometer readings.
Figuring out how to get sample loops to mix on the fly at 44.1kHz was a little tricky, but it ended up not being much code (you can download it from http://buglabs.net/applications/Phunky if you’re interested).
Implementation
When the application starts it creates an instance of theSampleStream
class, which extends InputStream
, and starts playing it via IModuleAudioPlayer.play()
; as far as IModuleAudioPlayer
is aware, SampleStream
is just a stream of data from a Wave file.The main loop in
PhunkyApplication.run()
then runs forever, grabbing samples from the AccelerometerSampleStream
as fast as it can, and passing the X, Y and Z values over to the SampleStream
instance.Since
SampleStream
extends InputStream
, IModuleAudioPlayer
is constantly grabbing sample data from it. SampleStream
includes a faked Wave file RIFF header. It outputs that header first, and then outputs the mixed sample data from then on.SampleStream
owns nine instances of the Sample
class, which also extends InputStream
. Each instance of Sample
wraps a raw sample data file that comes with the application (more on them later).The nine
Sample
instances are assigned three-to-an-axis. Thus whenever SampleStream
is asked for data to be played (after the fake Wave header has been output), it looks at the X, Y and Z values it was last given by the main application loop, uses those values to choose a Sample
instance for each axis, then reads a sample from each and mixes them together.Because the reading of accelerometer data is decoupled from the generation of the mixed sample data, there’s no concern about stuttering:
SampleStream
will simply continue mixing the same 3 Sample
streams together until it gets updated accelerometer data.That sounds like a lot of code, but it really isn’t: here’s the entire
SampleStream.read()
method (apologies for the formatting; I need to change the width of this column I really do…):public int read() throws IOException {
int sample = 0;
if (tearDownRequested) {
System.out.println("Returning -1 to make audio stream appear done");
sample = -1;
}
else if (byteCount < riffHeader.length) {
// return the next byte of our canned header
sample = riffHeader[byteCount];
}
else {
// return the most recently computed sample based on the update() data
// pick which samples to combine to create out output sample
// based on the accelerometer values we have been given
if ((byteCount % 4) == 0) {
// we return sample data 8 bits at a time, but the samples
// themselves are 16 bits each, and in stereo so there are
// two samples that go together, so we mustn't switch streams
// in the middle of a sample or we'll be out of sync (returning
// half of one sample and half of the next). We can use the same
// byteCount as we used for the header because the header has an
// even number of bytes
xIn = chooseInput(x, xLowThreshold, xHighThreshold, x1, x2, x3);
yIn = chooseInput(y, yLowThreshold, yHighThreshold, y1, y2, y3);
zIn = chooseInput(z, zLowThreshold, zHighThreshold, z1, z2, z3);
}
try {
sample = xIn.read() + yIn.read() + zIn.read();
}
catch (IOException e) {
// ignore it as it shouldn't happen with our InputStreams anyway
}
}
++byteCount;
return sample;
}
Because
IModuleAudioPlayer.play()
sees SampleStream
as a simple InputStream
, and SampleStream
mixes together some InputStream
s (the Sample
instances) the code can be tweaked very easily to do different things: for example you could mix in another InputStream
from the microphone, replace an axis with streams chosen by buttons, or any number of variations, without much of the code needing to be modified.Generating the Samples
At first I tried using simple samples that I generated using thetones
command-line utility, but they weren’t all that exciting. So I played around with the excellent Hydrogen drum machine application instead. I made loops from existing samples in Hygrogen (claves, cowbell, floor tom, etc.) and exported them as raw 44.1kHz 16-bit stereo, little-endian, sample files. These are a lot more fun (at least for a few minutes) and sound pretty darn good through the BUGsound.More Cowbell!
Here’s a video showing Phunky in action:
Bug Labs Community Site
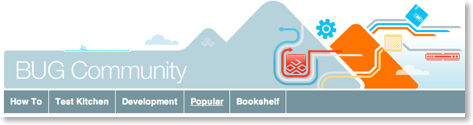
Bug Labs just made their community site official. You can find it at:
http://community.buglabs.net/
I’ve posted a few things up there so far. I think it’s a neat idea: letting the community share what they’re working on and what they’ve figured out. You could sort of do that on the forums, but the new site is much more feature-rich. It isn’t even restricted to projects directly involving the BUG; here’s an example.
Disclaimer: I’m doing some consultancy for Bug Labs now; but that is because I like the BUG and the folks at Bug Labs, not why I like it/them. :-)
BUG Unboxing
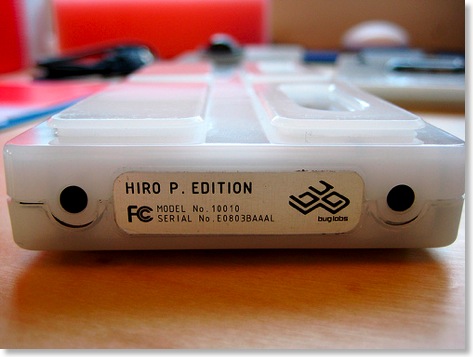
My BUGbundle arrived yesterday. I put a set of unboxing pictures of it on Flickr. I pinged Engadget after I posted them, since they've been following the BUG for a while. I didn't really expect them to pick it up, but they did. My Flickr stats will be forever skewed ;-)
UPDATE:
Yup, 30000+ views of those Bug pictures in the last day and a half: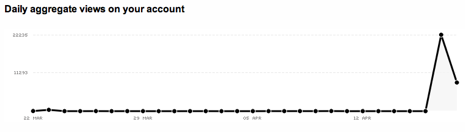
BugLabs update
Back in November, they had an "application cook-off" that I co-won with koolatron. Jeremy, the BugLabs marketing guy had said "prize details forthcoming" back then, and I'd pretty much forgotten about it until I watched the Engadget video with Jeremy at CES. I sent him a friendly reminder after he got back from CES and he wrote back with:
Was waiting til Monday to do this, but hey, it's the weekend, why not?
![]()
We're going to be sending two BUGbundles out, one for you, one for Koolatron. So you get the BUGbase and all four shipping modules, and we'll send you the "von Hippel" module when it's ready!
Sorry for the long, long wait - we had planned to do this some time ago, but the snag we hit early on in manufacturing basically nixed our whole approach. But, we're back up and running now...
I was amazed. The kit's early adopter price is $549, so they are being exceedingly generous. I didn't spend nearly enough time with the bug to warrant that, but now that I have actual hardware coming I will need to get back into working on some of my apps.
I took a gander over to bugnet and saw that, now that the beta is open, the apps are getting a lot more attention. One person even commented on mine about a typo; in this case one that was in the SDK last time I had compiled the app, but which has since been fixed by BugLabs. In the closed beta there were a lot of crickets chirping after the initial excitement, so It's nice to see the community around the bug starting to come alive.
I have to say, I'm excited to be getting a bug. With my intention to be more frugal in 2008, I had written off my chances of getting one to play with, but now I'm going to get one guilt-free! Maybe I will finally get round to making that GpsLogger do some actual logging.
BugLabs Beta Now Open
BugLabs update
We did get an update from Jeremy (the marketing guy) update the beta program back on November 20th:
Hi there - we know that it's gotten a little quiet around here and we want to apologize. We also want to give you an update (overdue!) so you don't think something is amiss.
First - BIG thanks to everyone whose been involved in our beta program. We've learned a ton from you and are applying it to our software and hardware. We would not be where we are right now without you. Please keep pounding. There's more fun stuff on the way.
Second, when we initiated this beta program we got a great response from everyone - applicants, selected testers, etc. Many people jumped in and started exploring, coding and building things. Others hung back and waited to see what unfolded. And the truth is, not very many did. It became clear to us pretty quickly that we were asking a lot of you. Without hardware to play with and more module types to mash up, folks ran out of energy. We intended to get more resources into the mix but we weren't able to because everyone's working hard on getting product out the door. Since then we've been hiring and I'm happy to say we will be making hardware available soon too!
We are a few defects away from moving out of closed beta and into an open beta of the BUG SDK and BUGnet. And as I mentioned above, our hardware is fully up and operational (although we can't say there's no defects just yet!). Needless to say, we're pretty excited about getting to this point!
In December, we'll be bringing the first batch of BUGbases and BUGmodules to market. These initial BUGbase units will be limited production v0.9 hardware. We're giving them that designation for a couple reasons. We decided to change the design of the front panel a little bit and will not be able to get that change into these first units before the end of the year. Same thing for the wifi chip set. We will not be able to incorporate our new wifi design into BUGbase before year end. So rather than delay shipment, we've decided to go into limited production. These units are functionally identical to what our final production units will be (with the only exception being wifi). All our BUGmodules will be v1.0 production and will be fully compatible with both our limited production and full production BUGbases.
Between now and the open beta, you are welcome to continue to use BUGnet, the SDK, and participate in the forums. There will be at least one update to the SDK in the meantime. As we have reached this great milestone, we invited some of our blogger friends to come take a look at working hardware, here's a few videos (Gizmodo, TechCrunch, Silicon Alley Insider) that show it off. If any of you'd like to come by the office, please let us know, we'd love to meet you in person. For those of you in the SF Bay Area, we're having a meetup on November 29th and we hope you can stop by!
Thanks again,
Jeremy
I asked a couple of follow-up questions (sorry for the image c&p):
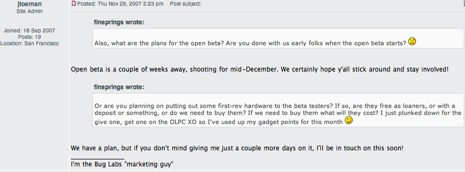
I'll post back when he lets us know what the plan is. They demoed some units running Qtopia to the press, but not anything running within the BUG framework. It's going to be interesting to see how the BUG SDK fits into the rest of the device's infrastructure.
BugLabs: Pictures of the real thing
I noticed tonight that one of the press images, which is labelled "Community Applications Image", is the one that they used on the t-shirt they sent me, and it includes the name of the first app that I posted: GpsLogger (which still doesn't log; I ought to get on that, huh).


BugLabs: Location-Aware To-do List Manager
I'm trying to do my part anyway (this is fun for me) and so I posted the fifth community app tonight. I found time last night to put together a first pass at a location-aware to-do list manager application and cleaned up the GUI some tonight. It's far from pretty, but it has several (canned for now) to-do lists, each with a name, related location and list of items. On the VirtualBUG's LCD modules, it looks like this for now:
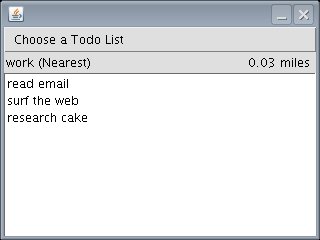
("Choose a Todo List" is a menu)
Once you've plugged the LCD and GPS modules into the bug, it will by default show you the to-do list whose location is nearest to your current position. I made it so that you could also explicitly select a list from a menu though. I posted it as-is, but I need to come up with a way of actually creating and working with lists. The bug doesn't have a keyboard, but an on-screen one could be created, iPhone-style. I've also considered exposing the lists via a servlet, but that would only be helpful if you had a 'real' computer to-hand; ideally you could work with lists directly on the bug. Another possibility in the less-than-ideal camp is to make it sync with web-based to-do list sites such as Remember The Milk or Ta Da Lists. I posted my thoughts on the forum, so I'll see what the buglabs folks and the other beta-testers think before going much further. I certainly don't fancy trying to create the on-screen keyboard myself in PhoneME's AWT.
BugLabs t-shirt
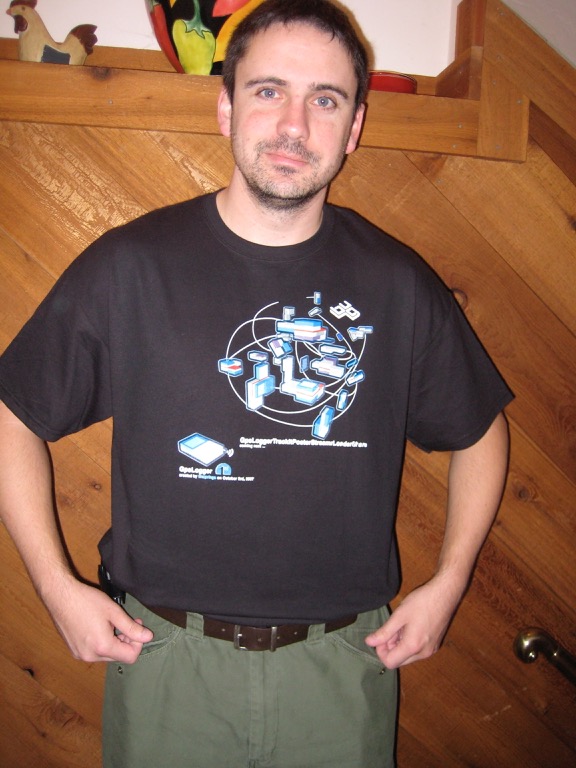
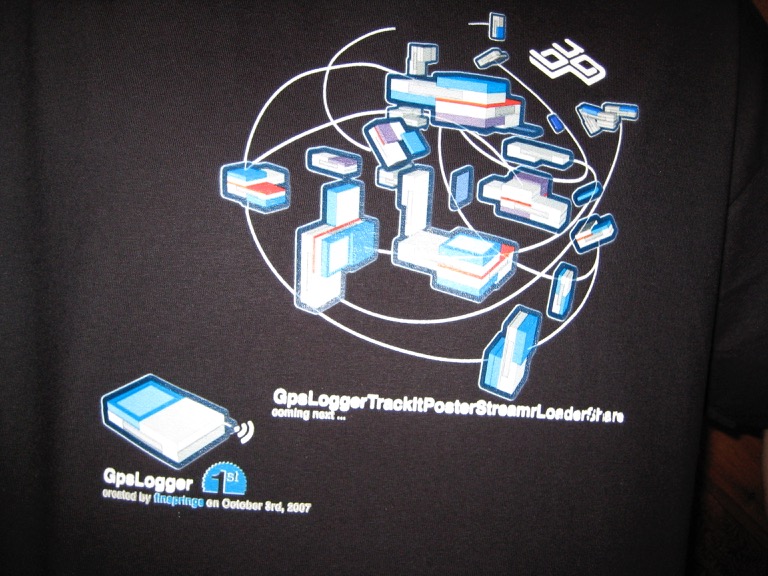
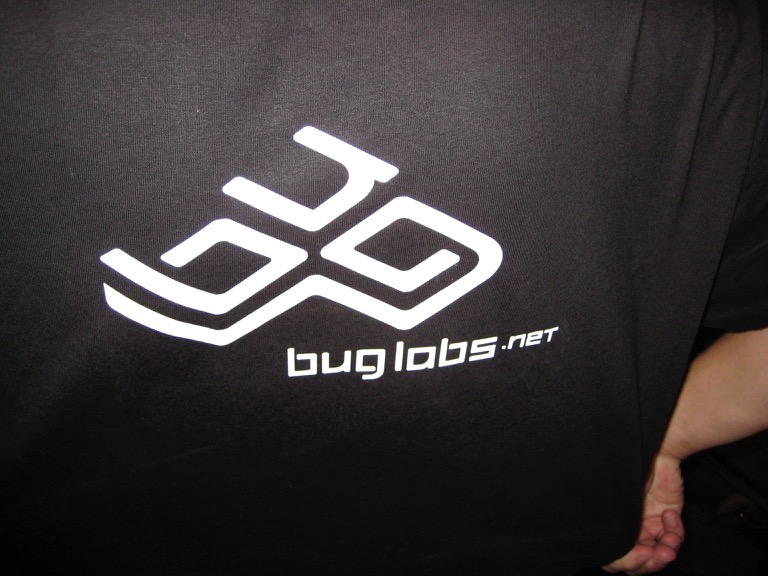
BugLabs: New SDK
One of the other beta testers, koolatron, took the simple GpsLogger app I pushed out and came up with a much nicer version, complete with a properly centered google tile and button-based zooming. I haven't had much time to spend on the Bug, between the release march at work and DIY stuff at home. I'm looking forward to messing around with it again, but it probably won't be this week.
Oh, I got a very nice email from the BugLabs marketing guy Jeremy:
Dave,
Just wanted to let you know your first prize is coming at ya. Please know it might show up in a fairly generic package, but it's unique and we spent a lot of time making something we thought had the right amount of personal touch. Hope you receive it as fondly as we sent it, and please let me know when it arrives - sometime next week I believe.
Best,
Jeremy
I'll post back when it arrives.
BugLabs: Second App "BUGer"
Hi, Now that you've had some time playing around in a fairly open context, we'd like to try a structured project where everyone is working on the same task, and see how the results play out.
The first project is to use the BUGbase and the motion detector module to build a home security system. This is a pretty straightforward project (in our estimation) and shouldn't take too long if you're already up to speed on the platform. For those of you new to the environment, it should be a good way to learn the basics of BUG.
Here's the "specifications" of the home security system: On any detected motion, the system should log an alert with the current date/time stamp. The alert log should be accessible via a Web interface.
Yup, it's that basic. For a little extra help, here are the basic steps to build the system: 1. Create a new BUG application, in the creation wizard select the IMotionSubject service.
2. Register a listener for callbacks to when motion is detected. Create and append to a file and log the date and time of occurence.
3. Create a menu item for the application that allows for the logging to be turned on and off.
4. Create a servlet that retrieves the log data from the log file and presents it to a web client.
5. Confirm that your application works by triggering motion events from the command line.
Once you are done, upload the app to BUGnet. If you need any help with the project, please let us know.
We also have a few ideas for those of you who want to push your app "to 11" (although you can be as creative as you want!), here are some of ours: * When an event happens, send an SMS * Send an email report at the end of every day * Double gold star project: also integrate with the camera, and make a picture available with each event. * something else???
Thanks, we'd love to see this wrapped up in a week if possible!
Best,
Jeremy
_________________
I'm the Bug Labs "marketing guy"
I had a bit of spare time on Sunday night so I put together "BUGer" that does pretty much what Jeremy was asking for. I added in the camera stuff too, so BUGer takes a picture on each motion event. However the servlet container in the VirtualBUG doesn't yet support static resources, so the image can't be displayed on the webpage. It's there for when it can though. If I find time I may look at putting in the email part, but the PhoneME JME doesn't have email stuff in it that I could see, and scraping SMTP seems tedious, so I may not. I'm also not sure how you would do SMS unless you did it as email-to-your-cell-phone, which is just email from the BUG's perspective, since the SMS part is done by the carrier's email server.
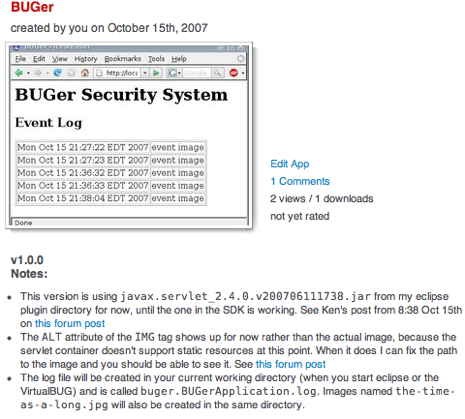
BugLabs: My first app came first...and last
One of the other testers is working on a new and improved version of my GpsLogger application. I'm looking forward to seeing what he comes up with. I bumped my version to 1.0.3 last night after factoring out the utility classes to a separate package and doing some general cleanup and documentation. I'm thinking about doing some webcam stuff with it next. There's a sample app that posts images to Flickr, but I'd like to do it more like a regular webcam. It would be pretty cool to update a picture every few minutes while you're out and about (assuming of course you have municipal WiFi...).
BugLabs: My GpsLogger gets slightly less lame
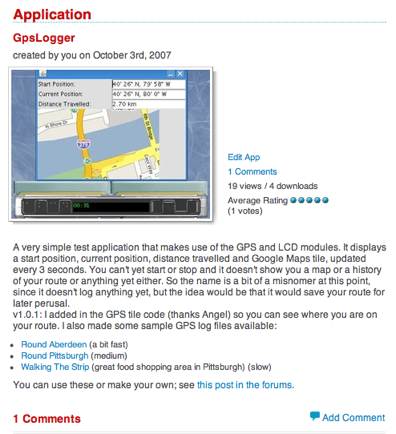
BugLabs New Rendering
BugLabs Update: My first app
They had their first webinar with the beta testers on Thursday, but we're in the final throes of getting a release completed at work, so I couldn't attend. They've also initiated an application cook-off where they judge tester-created applications and award some prizes. I doubt my humble offering will make it too far in the process, but it was at least the first user-generated app uploaded to the site.
Right, I best be off for now; gotta remote into a customer site and do an upgrade soon. I'll leave you with the entry for my first app:
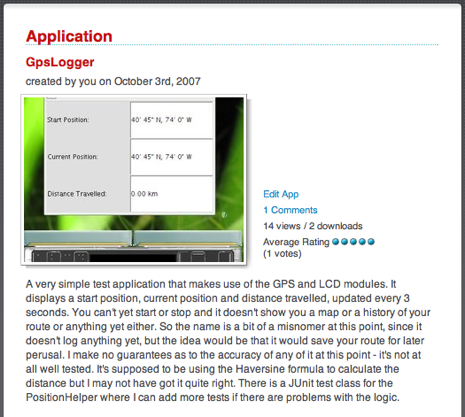
BugLabs Beta is a go!
We're not under a formal NDA or anything, but we've been asked not to disclose URLs for the various components of the beta program, and so on, which is fair enough:
They have bug tracking, forums and a WiKi already up and running, and they made an SDK available. The SDK includes VirtualBug: an emulator for the hardware, since hardware isn't available at this stage.By the way, please keep these links to yourself, they should all be considered confidential to the beta program. You are welcome to blog about, chat about, Twitter about, text about, email about, or send smoke signals about the beta program, but please don't share the links or SDK.
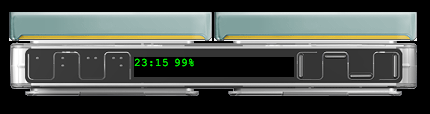
They built their SDK on top of Eclipse and I have to say it's pretty slick for things being so early. The instructions to get everything up and running are also unusually polished for a beta program.
There are a few sample applications that do real things, like post pictures to Flickr with geo-tagging. Some things are still necessarily in the mock-up stage until the hardware is ready, like the camera module (which pulls pictures from file rather than an actual camera) and the motion sensor (which 'detects' motion by you typing the word 'motion' into the Eclipse console).
Still, I'm very impressed at how it looks at this point. The few questions I've had have been replied to promptly in the forums and the folks at BugLabs all seem very helpful.
I'm still getting my feet wet in the SDKs, but I'll post updates as I make progress.
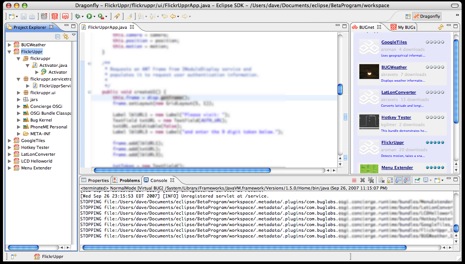